Flight plan - HALO-20240912a#
ec_under ec_track c_mid c_southCrew#
Job |
Name |
---|---|
PI |
Georgios Dekoutsidis |
WALES |
Sabrina Zehlau |
HAMP |
Janina Bömeke |
Dropsondes |
Jakob Deutloff |
Smart/VELOX |
Michael Schäfer |
SpecMACS |
Anja Stallmach |
Flight Documentation |
Felix Ament |
Ground contact |
Manfred Wendisch |
Flight plan#
Show code cell source
from datetime import datetime
import orcestra.sat
from orcestra.flightplan import bco, point_on_track, LatLon, IntoCircle, FlightPlan
# Some fixed coordinates
lon_min, lon_max, lat_min, lat_max = -65, -5, -5, 25
airport = bco
radius = 72e3*1.852
# Define dates for flight
flight_time = datetime(2024, 9, 12, 12, 0, 0)
flight_index = f"HALO-{flight_time.strftime('%Y%m%d')}a"
# Load satellite tracks
ec_fcst_time = "2024-09-11"
ec_track = orcestra.sat.SattrackLoader("EARTHCARE", ec_fcst_time, kind="PRE",roi="BARBADOS") \
.get_track_for_day(f"{flight_time:%Y-%m-%d}")\
.sel(time=slice(f"{flight_time:%Y-%m-%d} 14:00", None))
# Create elements of track
ec_track5 = ec_track.assign(lon=lambda ds: ds.lon + 3.5)
c_northeast = point_on_track(ec_track5,lat= 12.00).assign(label = "c_northeast")
c_southwest = point_on_track(ec_track,lat= 11.00).assign(label = "c_southwest")
c_southeast = point_on_track(ec_track5,lat= 9.00).assign(label = "c_southeast")
c_northwest = point_on_track(ec_track,lat= 14.00).assign(label = "c_northwest")
ec_north = point_on_track(ec_track,lat= 14.50).assign(label = "ec_north")
ec_south = point_on_track(ec_track,lat= c_southwest.lat - 1.5).assign(label = "ec_south")
ec_under = point_on_track(ec_track, lat= 12.00, with_time=True).assign(label = "ec_under", note = "meet EarthCARE")
ec_under_north = point_on_track(ec_track, lat= 13.00).assign(label = "ec_under_n", note = "EC align north")
ec_under_south = point_on_track(ec_track, lat= 11.00).assign(label = "ec_under_s", note = "EC align south")
# Define Flight Paths
waypoints = [
airport.assign(fl=0),
#c_southwest.assign(fl=410),
IntoCircle(c_southeast.assign(fl=410), radius, 330, enter= 0),
IntoCircle(c_northeast.assign(fl=430), radius, -360, enter = 180),
IntoCircle(c_northwest.assign(fl=430), radius, -360, enter = 180),
c_northwest.towards(c_southwest, distance = -radius).assign(fl = 450, label = "ec_north"),
ec_under_north.assign(fl=450),
ec_under.assign(fl=450),
ec_under_south.assign(fl=450),
ec_south.assign(fl=450),
IntoCircle(c_southwest.assign(fl=450), radius, 360),
airport.assign(fl=0),
]
#point_on_track(ec_track, lat=c_mid.towards(c_south, distance = radius).lat).assign(fl=450, label="fl_change"),
#c_northwest.assign(fl=450),
# ec_north.assign(fl=450),
# Crew
crew = {'Mission PI': 'Georgios Dekoutsidis',
'DropSondes': 'Jakob Deutloff',
'HAMP': 'Janina Bömeke',
'SMART/VELOX': 'Michael Schäfer',
'SpecMACS': 'Anja Stallmach',
'WALES' : 'Sabrina Zehlau',
'Flight Documentation': 'Felix Ament',
'Ground Support': 'Manfred Wendisch',
}
# Plan
plan = FlightPlan(waypoints, flight_index, crew=crew, aircraft="HALO")
print(f"Flight index: {plan.flight_id}")
print(f"Take-off: {plan.takeoff_time:%H:%M %Z}\nLanding: {plan.landing_time:%H:%M %Z}\n")
#pace_track = orcestra.sat.pace_track_loader() \
# .get_track_for_day(f"{flight_time:%Y-%m-%d}")
#pace_track = pace_track.where(
# (pace_track.lat >lat_min)&
# (pace_track.lat <lat_max)&
# (pace_track.lon >-60)&
#(pace_track.lon <-30),
#drop = True) \
# .sel(time=slice(f"{flight_time:%Y-%m-%d} 11:00", f"{flight_time:%Y-%m-%d} 21:00"))
Flight index: HALO-20240912a
Take-off: 11:40 UTC
Landing: 20:06 UTC
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/sat.py:183: UserWarning: You are using an old forecast (issued on 2024-09-11) for EARTHCARE on 2024-09-12! The newest forecast issued so far was issued on 2024-09-12. It's a PRE forecast.
warnings.warn(
Show code cell source
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import easygems.healpix as egh
import intake
from orcestra.flightplan import plot_cwv, plot_path
forecast_overlay = True
plt.figure(figsize = (14, 8))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([lon_min, lon_max, lat_min, lat_max], crs=ccrs.PlateCarree())
ax.coastlines(alpha=1.0)
ax.gridlines(draw_labels=True, dms=True, x_inline=False, y_inline=False, alpha = 0.25)
if (forecast_overlay):
ifs_fcst_time = datetime(2024, 9, 11, 0, 0, 0)
ds = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml").HIFS(datetime = ifs_fcst_time).to_dask().pipe(egh.attach_coords)
cwv_flight_time = ds["tcwv"].sel(time=flight_time, method = "nearest")
plot_cwv(cwv_flight_time, levels = [48.0, 50.0, 52.0, 54.0, 56.0, 58.0, 60.0], ax=ax)
plt.title(f"{flight_time}\n(CWV forecast issued on {ifs_fcst_time})")
plt.plot(ec_track.lon, ec_track.lat, c='k', ls='dotted')
plot_path(plan, ax, color="C1")
#plt.plot(pace_track.lon, pace_track.lat, c='k', ls ='dashed', label = 'PACE')
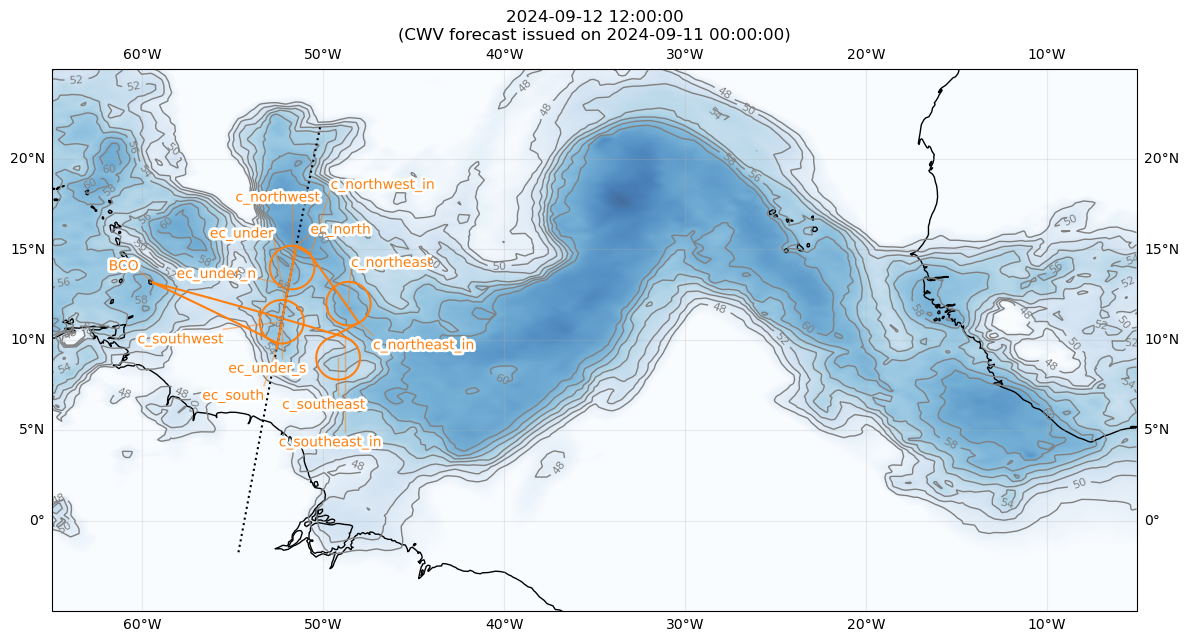
Show code cell source
from orcestra.flightplan import vertical_preview
vertical_preview(waypoints)
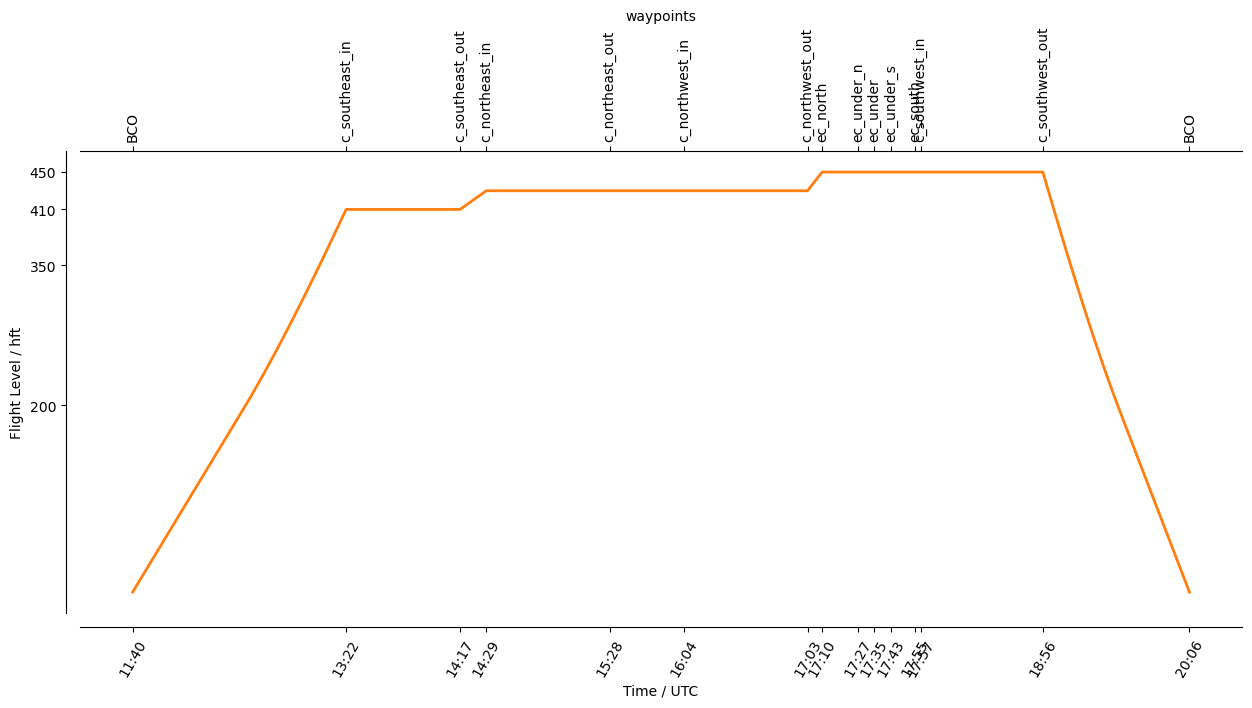
Show code cell source
plan.show_details()
plan.export()
Detailed Overview:
BCO N13 09.76, W059 25.72, FL000, 11:40:23 UTC,
to (helper) c_southeast_in N10 09.15, W048 49.49, FL410, 13:22:36 UTC, upcoming circle will be entered here
circle around c_southeast N09 00.00, W049 10.88, FL410, 13:22:36 UTC - 14:17:12 UTC, radius: 72 nm, 330° CW, enter from north
to (helper) c_northeast_in N10 56.37, W048 01.73, FL430, 14:29:46 UTC, upcoming circle will be entered here
circle around c_northeast N12 00.00, W048 36.60, FL430, 14:29:46 UTC - 15:28:47 UTC, radius: 72 nm, 360° CCW, enter from south east
to (helper) c_northwest_in N14 41.99, W050 43.08, FL430, 16:04:35 UTC, upcoming circle will be entered here
circle around c_northwest N14 00.00, W051 43.47, FL430, 16:04:35 UTC - 17:03:36 UTC, radius: 72 nm, 360° CCW, enter from north east
to ec_north N15 11.03, W051 29.56, FL450, 17:10:33 UTC,
to ec_under_n N13 00.00, W051 55.06, FL450, 17:27:45 UTC, EC align north
to ec_under N12 00.00, W052 06.60, FL450, 17:35:37 UTC, meet EarthCARE
to ec_under_s N11 00.00, W052 18.08, FL450, 17:43:29 UTC, EC align south
to ec_south N09 30.00, W052 35.20, FL450, 17:55:17 UTC,
circle around c_southwest N11 00.00, W052 18.08, FL450, 17:57:46 UTC - 18:56:19 UTC, radius: 72 nm, 360° CW, enter from south
to BCO N13 09.76, W059 25.72, FL000, 20:06:31 UTC,
plan.duration
datetime.timedelta(seconds=30367, microseconds=711189)