Flight plan - HALO-20240907a#
ec_under ec_track c_north c_mid c_southCrew#
The flight is planned to take off at 2024-09-07 12:51:06+00:00.
Job |
Name |
---|---|
PI |
Bjorn Stevens |
WALES |
Georgios Dekoutsidis |
HAMP |
Clara Bayley |
Dropsondes |
Helene Glöckner |
Smart/VELOX |
Michael Schäfer |
SpecMACS |
Anja Stallmach |
Flight Documentation |
Daniel Rowe |
Ground contact |
Chavez Pope |
Flight plan#
Show code cell source
from dataclasses import asdict
from datetime import datetime
import orcestra.sat
from orcestra.flightplan import bco, sal, mindelo, point_on_track, LatLon, IntoCircle, FlightPlan
# Some fixed coordinates
lon_min, lon_max, lat_min, lat_max = -65, -5, -5, 25
airport = bco
radius = 72e3*1.852
# Define dates for flight
flight_time = datetime(2024, 9, 7, 12, 0, 0)
flight_index = f"HALO-{flight_time.strftime('%Y%m%d')}a"
# Load satellite tracks
ec_fcst_time = "2024-09-06"
ec_track = orcestra.sat.SattrackLoader("EARTHCARE", ec_fcst_time, kind="PRE",roi="BARBADOS") \
.get_track_for_day(f"{flight_time:%Y-%m-%d}")\
.sel(time=slice(f"{flight_time:%Y-%m-%d} 14:00", None))
# Create elements of track
c_south = point_on_track(ec_track,lat= 7.00).assign(label = "c_south")
c_mid = point_on_track(ec_track,lat= 10.25).assign(label = "c_mid")
c_north = point_on_track(ec_track,lat= 13.50).assign(label = "c_north")
c_wait = c_south.towards(c_mid,distance=radius*0.75).assign(label = "c_wait")
join = c_south.towards(c_mid,distance=radius).assign(label = "join")
c_out = c_north.towards(c_mid,distance=radius).assign(label = "c_out")
ec_north = point_on_track(ec_track,lat= 15.00).assign(label = "ec_north")
xlat = c_south.towards(c_mid,distance=-radius).lat
ec_south = point_on_track(ec_track,lat= xlat).assign(label = "ec_south")
ec_mid = ec_north.towards(ec_south).assign(label = "ec_mid")
ec_turn = ec_mid.towards(ec_south,fraction=2/3.).assign(label = "ec_turn")
xlat = c_mid.towards(c_north,fraction=1/6).lat
ec_under = point_on_track(ec_track, lat=xlat, with_time=True).assign(label = "ec_under", note = "meet EarthCARE")
# Additional Waypoints
extra_waypoints = []
# Define Flight Paths
waypoints = [
airport.assign(fl=0),
join.assign(fl=410),
IntoCircle(c_wait.assign(fl=410), radius/4, 360),
IntoCircle(c_south.assign(fl=410), radius,360),
join.assign(fl=410),
IntoCircle(c_mid.assign(fl=430), radius, -360,enter=-90),
c_mid.assign(fl=450),
ec_under.assign(fl=450),
c_out.assign(fl=450),
IntoCircle(c_north.assign(fl=450), radius, -360),
airport.assign(fl=0),
]
# Crew
crew = {
'Mission PI': 'Bjorn Stevens',
'DropSondes': 'Helene Glöckner',
'HAMP': 'Clara Bayley',
'SMART/VELOX': 'Michael Schäfer',
'SpecMACS': 'Anja Stallmach',
'WALES' : 'Georgios Dekoutsidis',
'Flight Documentation': 'Daniel Rowe',
'Ground Support': 'Chavez Pope'
}
# Plan
plan = FlightPlan(waypoints, flight_index, extra_waypoints=extra_waypoints, crew=crew)
print(f"Flight index: {plan.flight_id}")
print(f"Take-off: {plan.takeoff_time:%H:%M %Z}\nLanding: {plan.landing_time:%H:%M %Z}\n")
Flight index: HALO-20240907a
Take-off: 12:51 UTC
Landing: 20:16 UTC
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/sat.py:195: UserWarning: You are using an old forecast (issued on 2024-09-06) for EARTHCARE on 2024-09-07! The newest forecast issued so far was issued on 2024-09-07. It's a PRE forecast.
warnings.warn(
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/flightplan.py:214: FutureWarning: The aircraft attribute of this FlightPlan is not set, but it's required for aircraft performance calculations. Currently, the code will proceed using the default aircraft performance, but the code may break in future.
warn(
Show code cell source
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import easygems.healpix as egh
import intake
from orcestra.flightplan import plot_cwv, plot_path
forecast_overlay = True
plt.figure(figsize = (14, 8))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([lon_min, lon_max, lat_min, lat_max], crs=ccrs.PlateCarree())
ax.coastlines(alpha=1.0)
ax.gridlines(draw_labels=True, dms=True, x_inline=False, y_inline=False, alpha = 0.25)
if (forecast_overlay):
ifs_fcst_time = datetime(2024, 9, 4, 0, 0, 0)
ds = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml").HIFS(datetime = ifs_fcst_time).to_dask().pipe(egh.attach_coords)
cwv_flight_time = ds["tcwv"].sel(time=flight_time, method = "nearest")
plot_cwv(cwv_flight_time, levels = [50.0, 60.0], ax=ax)
plt.title(f"{flight_time}\n(CWV forecast issued on {ifs_fcst_time})")
plt.plot(ec_track.lon, ec_track.lat, c='k', ls='dotted')
plot_path(plan, ax, color="C1")
if (False):
natal = LatLon(-5.795,-35.209439, label = "natal")
airways = [natal,sal]
plot_path(airways, ax, color="purple")

Show code cell source
from orcestra.flightplan import vertical_preview
vertical_preview(waypoints)
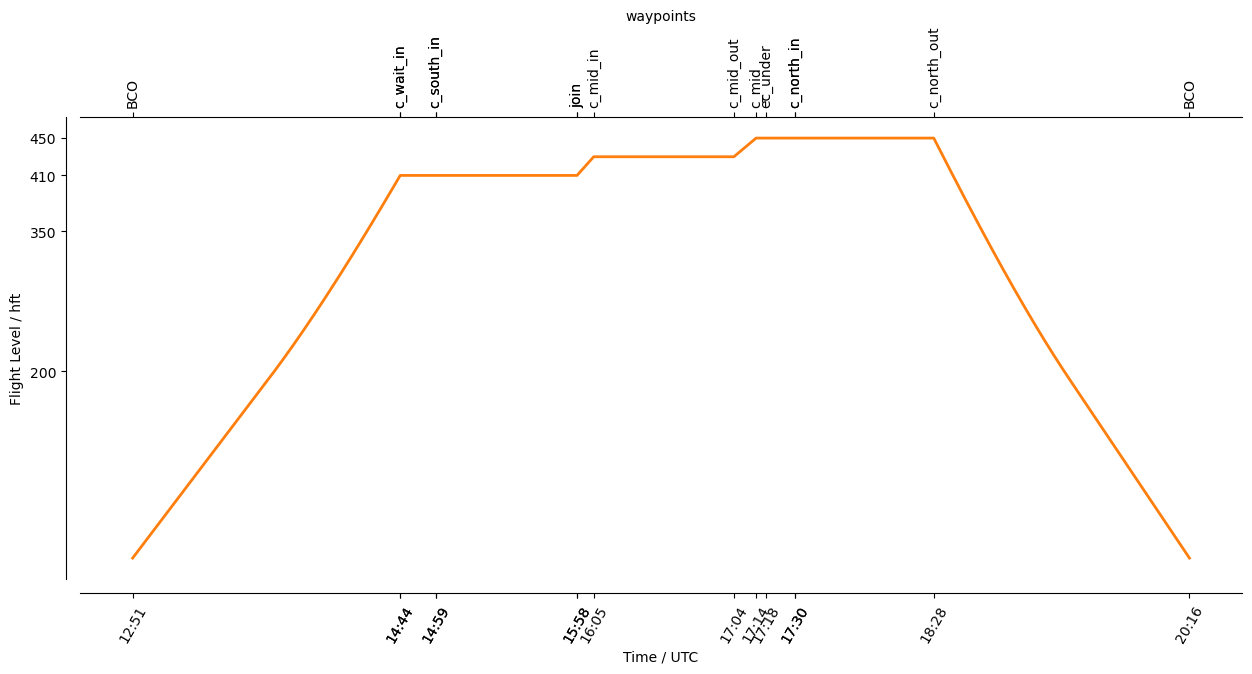
Show code cell source
plan.show_details()
plan.export()
Detailed Overview:
BCO N13 09.76, W059 25.72, FL000, 12:51:31 UTC,
to join N08 11.10, W048 23.92, FL410, 14:44:11 UTC,
circle around c_wait N07 53.33, W048 27.27, FL410, 14:44:11 UTC - 14:59:04 UTC, radius: 18 nm, 360° CW, enter from north
circle around c_south N07 00.00, W048 37.29, FL410, 14:59:04 UTC - 15:58:38 UTC, radius: 72 nm, 360° CW, enter from north
to join N08 11.10, W048 23.92, FL410, 15:58:38 UTC,
circle around c_mid N10 15.00, W048 00.42, FL430, 16:05:39 UTC - 17:04:40 UTC, radius: 72 nm, 360° CCW, enter from south
to c_mid N10 15.00, W048 00.42, FL450, 17:14:01 UTC,
to ec_under N10 47.51, W047 54.23, FL450, 17:18:17 UTC, meet EarthCARE
to c_out N12 18.95, W047 36.77, FL450, 17:30:16 UTC,
circle around c_north N13 30.00, W047 23.04, FL450, 17:30:16 UTC - 18:28:49 UTC, radius: 72 nm, 360° CCW, enter from south
to BCO N13 09.76, W059 25.72, FL000, 20:16:28 UTC,
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/flightplan.py:214: FutureWarning: The aircraft attribute of this FlightPlan is not set, but it's required for aircraft performance calculations. Currently, the code will proceed using the default aircraft performance, but the code may break in future.
warn(