Flight plan - HALO-20240903a#
ec_under ec_track c_north c_mid c_south c_atrCrew#
The flight is planned to take off at 2024-09-03 11:04:13+00:00.
Job |
Name |
---|---|
PI |
Bjorn Stevens |
WALES |
Tanya Bodenbach |
HAMP |
Clara Bayley |
Dropsondes |
Nina Robbins |
Smart/VELOX |
Sophie Rosenburg |
SpecMACS |
Zekican Demiralay |
Flight Documentation |
Marius Rixen |
Ground contact |
Karsten Peters |
Flight plan#
Show code cell source
from datetime import datetime
import orcestra.sat
from orcestra.flightplan import bco, sal, point_on_track, LatLon, IntoCircle, FlightPlan
# Some fixed coordinates
lon_min, lon_max, lat_min, lat_max = -65, -5, -5, 25
band = "east"
airport = sal if band == "east" else bco
radius = 72e3*1.852
atr_radius = 38e3*1.852
c_atr_nw = LatLon(17.433,-23.500, label = "c_atr")
c_atr_se = LatLon(16.080,-21.715, label = "c_atr")
# Define dates for flight
flight_time = datetime(2024, 9, 3, 12, 0, 0)
flight_index = f"HALO-{flight_time.strftime('%Y%m%d')}a"
# Load satellite tracks
ec_fcst_time = "2024-09-02"
ec_track = orcestra.sat.SattrackLoader("EARTHCARE", ec_fcst_time, kind="PRE") \
.get_track_for_day(f"{flight_time:%Y-%m-%d}")\
.sel(time=slice(f"{flight_time:%Y-%m-%d} 14:00", None))
# Create elements of track
ec_north = point_on_track(ec_track,lat= 14.75).assign(label = "ec_north")
ec_south = point_on_track(ec_track,lat= 2.00).assign(label = "ec_south")
c_north = point_on_track(ec_track,lat= 13.00).assign(label = "c_north")
c_south = point_on_track(ec_track,lat= 4.00).assign(label = "c_south")
c_mid = point_on_track(ec_track,lat=c_south.towards(c_north).lat).assign(label = "c_mid")
c_atr = LatLon(ec_north.lat+atr_radius/1852./60.,-22.1, label = "c_atr")
j_beg = LatLon(lat=ec_north.lat,lon=-25.).assign(label = "junction")
ec_under = point_on_track(ec_track, lat=c_mid.towards(c_north,fraction=2./5.).lat, with_time=True).assign(label = "ec_under", note = "meet EarthCARE, drop sonde")
# Additional Waypoints
x_cnn = point_on_track(ec_track,lat= 13.67).assign(label="x_cnn", note="Northern alternative center for c_north")
x_cns = point_on_track(ec_track,lat= 11.50).assign(label="x_cns", note="Southern alternative center for c_north")
x_cms = point_on_track(ec_track,lat= 7.75).assign(label="x_cms", note="Southern alternative center for c_mid")
x_xsd = c_south.towards(c_mid).assign(label="x_sonde", note="Position for an extra sonde heading south")
j_atr = c_atr.towards(sal,distance=-atr_radius).assign(label="j_atr", note = "ATR circle start and end")
extra_waypoints = [LatLon(17.5,-24.00).assign(label="x_atr", note="alternative ATR circle")]
# Define Flight Paths
waypoints = [
airport.assign(fl=0),
j_beg.assign(fl=390),
ec_north.assign(fl=410),
c_mid.assign(fl=410),
x_xsd.assign(fl=410),
IntoCircle(c_south.assign(fl=410), radius, -360),
x_cms.assign(fl=450).assign(note='might be to soon to rise to FL450, if so rise to FL430 and higher later'),
IntoCircle(c_mid.assign(fl=450), radius, -360),
ec_under.assign(fl=450),
x_cns.assign(fl=450),
IntoCircle(c_north.assign(fl=450), radius, -360,enter=90),
x_cnn.assign(fl=450),
ec_north.assign(fl=450),
j_beg.assign(fl=450),
j_atr.assign(fl=350),
IntoCircle(c_atr.assign(fl=350), atr_radius,-360),
airport.assign(fl=0),
]
# Crew
crew = {'Mission PI': 'Bjorn Stevens',
'DropSondes': 'Nina Robbins',
'HAMP': 'Clara Bayley',
'SMART/VELOX': 'Sophie Rosenburg',
'SpecMACS': 'Zekican Demiralay',
'WALES' : 'Tanja Bodenbach',
'Flight Documentation': 'Marius Rixen',
'Ground Support': 'Karsten Peters',
}
# Plan
plan = FlightPlan(waypoints, flight_index, extra_waypoints=extra_waypoints, crew=crew)
print(f"Flight index: {plan.flight_id}")
print(f"Take-off: {plan.takeoff_time:%H:%M %Z}\nLanding: {plan.landing_time:%H:%M %Z}\n")
Flight index: HALO-20240903a
Take-off: 11:04 UTC
Landing: 19:44 UTC
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/sat.py:183: UserWarning: You are using an old forecast (issued on 2024-09-02) for EARTHCARE on 2024-09-03! The newest forecast issued so far was issued on 2024-09-03. It's a RES forecast.
warnings.warn(
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/flightplan.py:205: FutureWarning: The aircraft attribute of this FlightPlan is not set, but it's required for aircraft performance calculations. Currently, the code will proceed using the default aircraft performance, but the code may break in future.
warn(
Show code cell source
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import easygems.healpix as egh
import intake
from orcestra.flightplan import plot_cwv, plot_path
plt.figure(figsize = (14, 8))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([lon_min, lon_max, lat_min, lat_max], crs=ccrs.PlateCarree())
ax.coastlines(alpha=1.0)
ax.gridlines(draw_labels=True, dms=True, x_inline=False, y_inline=False, alpha = 0.25)
ifs_fcst_time = datetime(2024, 9, 1, 0, 0, 0)
ds = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml").HIFS(datetime = ifs_fcst_time).to_dask().pipe(egh.attach_coords)
cwv_flight_time = ds["tcwv"].sel(time=flight_time, method = "nearest")
plot_cwv(cwv_flight_time, levels = [50.0, 60.0], ax=ax)
plt.title(f"{flight_time}\n(CWV forecast issued on {ifs_fcst_time})")
plt.plot(ec_track.lon, ec_track.lat, c='k', ls='dotted')
plot_path(plan, ax, color="C1")
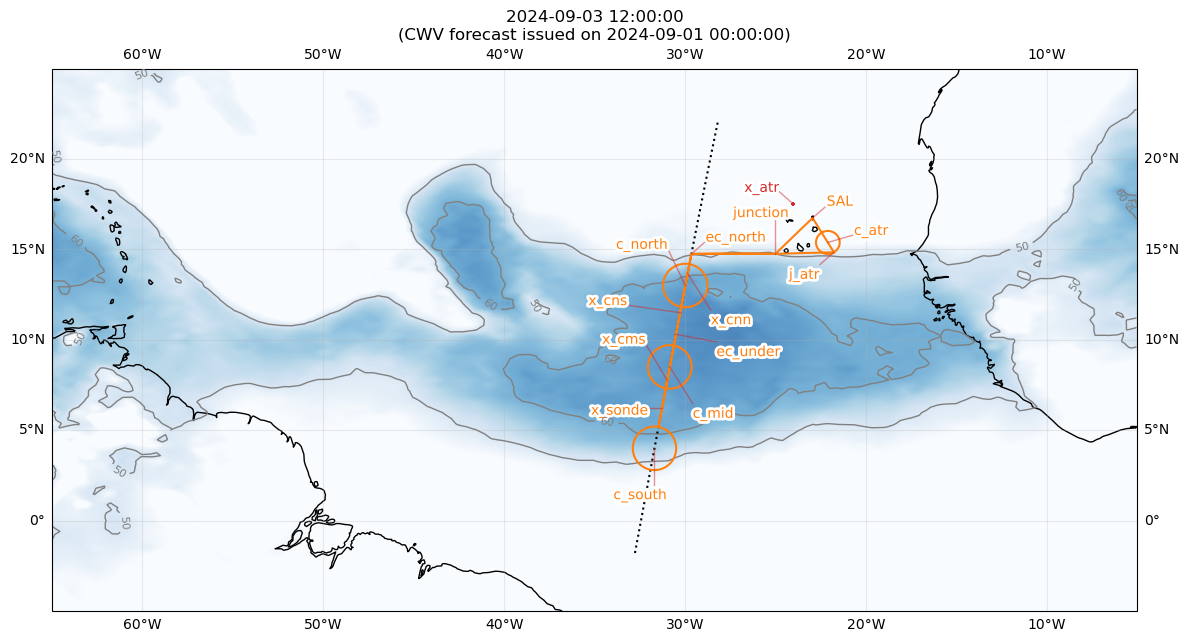
Show code cell source
from orcestra.flightplan import vertical_preview
vertical_preview(waypoints)
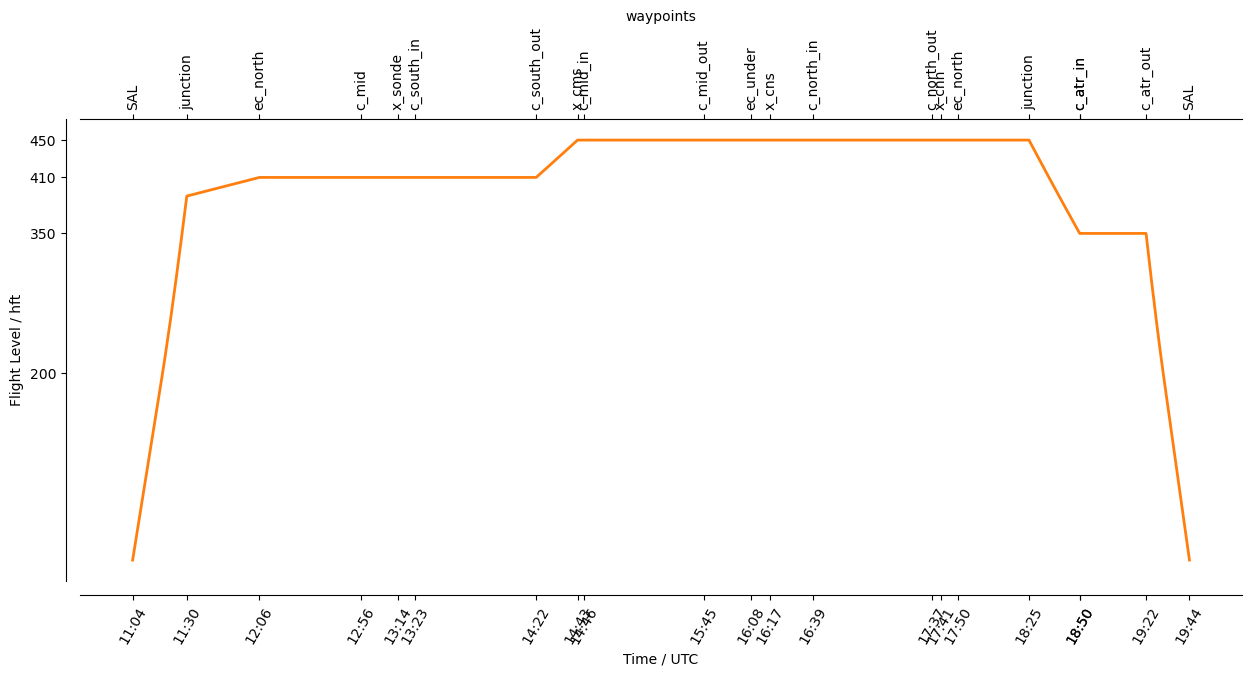
Show code cell source
plan.show_details()
plan.export()
Detailed Overview:
SAL N16 44.07, W022 56.64, FL000, 11:04:13 UTC,
to junction N14 45.00, W025 00.00, FL390, 11:30:52 UTC,
to ec_north N14 45.00, W029 38.68, FL410, 12:06:37 UTC,
to c_mid N08 30.09, W030 50.49, FL410, 12:56:38 UTC,
to x_sonde N06 15.06, W031 15.95, FL410, 13:14:39 UTC, Position for an extra sonde heading south
circle around c_south N04 00.00, W031 41.20, FL410, 13:23:10 UTC - 14:22:44 UTC, radius: 72 nm, 360° CCW, enter from north
to x_cms N07 45.00, W030 59.00, FL450, 14:43:04 UTC, might be to soon to rise to FL450, if so rise to FL430 and higher later
circle around c_mid N08 30.09, W030 50.49, FL450, 14:46:29 UTC - 15:45:01 UTC, radius: 72 nm, 360° CCW, enter from south
to ec_under N10 18.08, W030 30.02, FL450, 16:08:30 UTC, meet EarthCARE, drop sonde
to x_cns N11 30.00, W030 16.30, FL450, 16:17:56 UTC, Southern alternative center for c_north
circle around c_north N13 00.00, W029 59.02, FL450, 16:39:04 UTC - 17:37:36 UTC, radius: 72 nm, 360° CCW, enter from north, fly through circle before entering
to x_cnn N13 40.20, W029 51.26, FL450, 17:41:39 UTC, Northern alternative center for c_north
to ec_north N14 45.00, W029 38.68, FL450, 17:50:09 UTC,
to junction N14 45.00, W025 00.00, FL450, 18:25:06 UTC,
to j_atr N14 50.28, W021 45.79, FL350, 18:50:04 UTC, ATR circle start and end
circle around c_atr N15 23.00, W022 06.00, FL350, 18:50:04 UTC - 19:22:42 UTC, radius: 38 nm, 360° CCW, enter from south east
to SAL N16 44.07, W022 56.64, FL000, 19:44:03 UTC,
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/flightplan.py:205: FutureWarning: The aircraft attribute of this FlightPlan is not set, but it's required for aircraft performance calculations. Currently, the code will proceed using the default aircraft performance, but the code may break in future.
warn(