Flight plan - HALO-20240821a#
c_north c_mid c_south meteor c_atrCrew#
The flight is planned to take off at 2024-08-21 12:30:00+00:00.
Job |
Name |
---|---|
PI |
Cathy Hohenegger |
WALES |
Silke Gross |
HAMP |
Tobias Koelling |
Dropsondes |
Helene Gloeckner |
Smart/VELOX |
Kevin Wolf |
SpecMACS |
Zekican Demiralay |
Scientist |
Divya Praturi |
Ground contact |
Julia Windmiller |
Flight plan#
Show code cell source
from orcestra.flightplan import sal, bco, LatLon, IntoCircle, path_preview, plot_cwv
from datetime import datetime
import intake
import easygems.healpix as egh
cat = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml")
# Define dates for forecast initialization and flight
issued_time = datetime(2024, 8, 19, 0, 0, 0)
issued_time_str = issued_time.strftime('%Y-%m-%d')
flight_time = datetime(2024, 8, 21, 12, 0, 0)
flight_time_str = flight_time.strftime('%Y-%m-%d')
flight_index = f"HALO-{flight_time.strftime('%Y%m%d')}a"
print("Initalization date of IFS forecast: " + issued_time_str + "\nFlight date: " + flight_time_str + "\nFlight index: " + flight_index)
radius = 130e3
atr_radius = 70e3
airport=sal
mindelo = LatLon(lat=16.8778, lon=-24.995,label= "mindelo")
north_in = LatLon(lat=15.00, lon=-26.71,label= "north_in")
north_out = LatLon(lat=16.8778, lon=-26.71,label= "north_out")
south_tp = LatLon(lat=3., lon=-26.71,label= "south_tp")
c_extra = LatLon(13.7,-26.71, "c_extra")
c_south = LatLon(5.5, -26.71, "c_south")
c_north=LatLon(11.1,-26.71,"c_north")
c_mid=LatLon(8.3,-26.71,"c_mid")
leg_south = [
airport,
north_in,
c_extra,
c_north,
c_mid,
c_south,
south_tp
]
leg_circles = [
IntoCircle(c_south, radius, 360),
IntoCircle(c_mid, radius, 360),
IntoCircle(c_north, radius, 360),
IntoCircle(c_extra, radius, 360),
]
leg_home = [
north_out,
airport
]
path = leg_south+leg_circles+leg_home
cat = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml")
ds = cat.HIFS(datetime=issued_time).to_dask().pipe(egh.attach_coords)
cwv_flight_time = ds["tcwv"].sel(time=flight_time, method = "nearest")
ax = path_preview(path)
plot_cwv(cwv_flight_time)
Initalization date of IFS forecast: 2024-08-19
Flight date: 2024-08-21
Flight index: HALO-20240821a
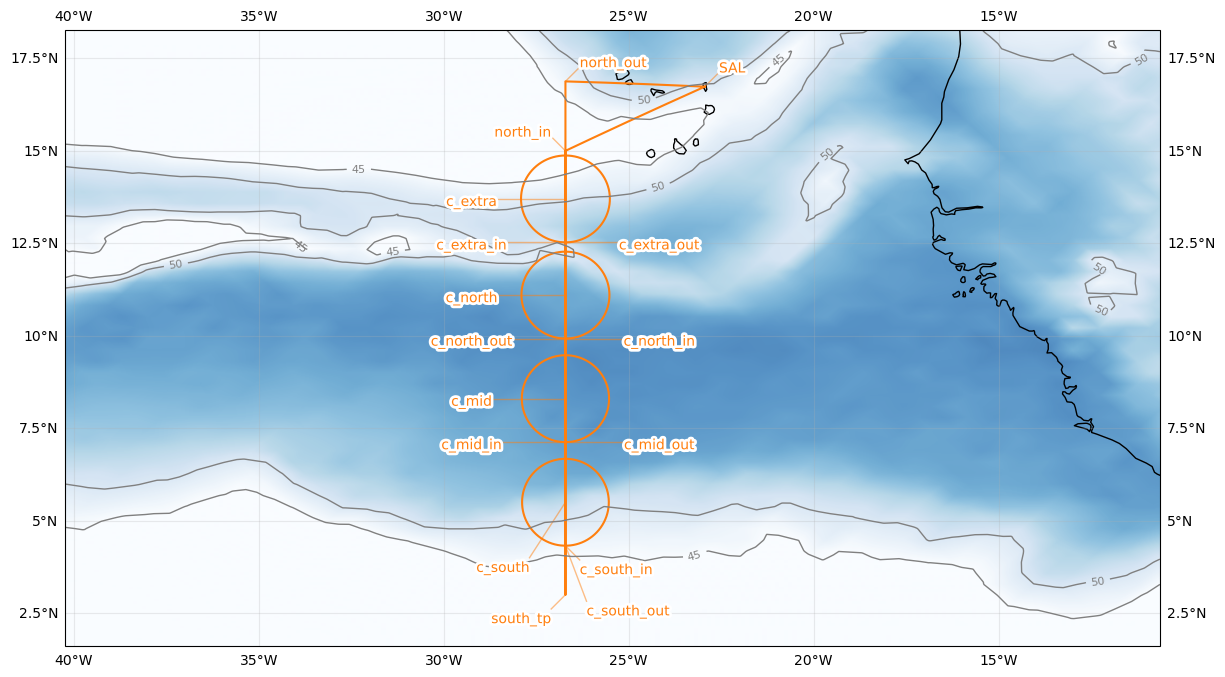
Show code cell source
import pandas as pd
from dataclasses import asdict
pd.DataFrame.from_records(map(asdict, [sal,north_in,c_extra,c_north,c_mid,c_south,south_tp,north_out])).set_index("label")
lat | lon | fl | time | note | |
---|---|---|---|---|---|
label | |||||
SAL | 16.734488 | -22.943974 | 0.0 | None | None |
north_in | 15.000000 | -26.710000 | NaN | None | None |
c_extra | 13.700000 | -26.710000 | NaN | None | None |
c_north | 11.100000 | -26.710000 | NaN | None | None |
c_mid | 8.300000 | -26.710000 | NaN | None | None |
c_south | 5.500000 | -26.710000 | NaN | None | None |
south_tp | 3.000000 | -26.710000 | NaN | None | None |
north_out | 16.877800 | -26.710000 | NaN | None | None |
Show code cell source
from orcestra.flightplan import export_flightplan
export_flightplan("HALO-20240821a", path)