Flight plan - HALO-20240923a#
ec_under c_mid c_southCrew#
Job |
Name |
---|---|
PI |
Theresa Mieslinger |
WALES |
Konstantin Krüger |
HAMP |
Janina Böhmeke |
Dropsondes |
Helene Glöckner |
Smart/VELOX |
Patrizia Schoch |
SpecMACS |
Anja Stallmach |
Flight Documentation |
Romain Fievet |
Ground contact |
Sebastian Ortega and Alessandro Savazzi |
Flight plan#
crew = {'Mission PI': 'Theresa Mieslinger',
'DropSondes': 'Helene Glöckner',
'HAMP': 'Janina Böhmeke',
'SMART/VELOX': 'Patrizia Schoch',
'SpecMACS': 'Anja Stallmach',
'WALES' : 'Konstantin Krüger',
'Flight Documentation': 'Romain Fievet',
'Ground Support': 'Sebastian Ortega and Alessandro Savazzi'
}
from datetime import datetime
import orcestra.sat
from orcestra.flightplan import tbpb, point_on_track, LatLon, IntoCircle, FlightPlan, aircraft_performance, vertical_preview
import topojson
import json
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import easygems.healpix as egh
import intake
from orcestra.flightplan import plot_cwv, plot_path, plot_fir
import numpy as np
General definitions#
flight_time = datetime(2024, 9, 23, 12, 0, 0)
flight_index = f"HALO-{flight_time.strftime('%Y%m%d')}a"
bco = LatLon(13.16263889, -59.42875000, "BCO", fl=0)
radius = 72e3*1.852
radius_meteor = 72e3
Load IFS forecast#
ifs_fcst_time = datetime(2024, 9, 22, 12, 0, 0)
ds = intake.open_catalog("https://tcodata.mpimet.mpg.de/internal.yaml").HIFS(datetime = ifs_fcst_time).to_dask().pipe(egh.attach_coords)
cwv_flight_time = ds["tcwv"].sel(time=flight_time, method = "nearest")
Load EC track#
ec_fcst_time = "2024-09-22"
ec_track = orcestra.sat.SattrackLoader("EARTHCARE", ec_fcst_time, kind="PRE",roi="BARBADOS") \
.get_track_for_day(f"{flight_time:%Y-%m-%d}")\
.sel(time=slice(f"{flight_time:%Y-%m-%d} 14:00", None))
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/sat.py:183: UserWarning: You are using an old forecast (issued on 2024-09-22) for EARTHCARE on 2024-09-23! The newest forecast issued so far was issued on 2024-09-23. It's a PRE forecast.
warnings.warn(
Meteor coordination after take-off#
c_climb = bco.assign(lon=bco.lon+0.61, label="c_climb", fl=150, note="circle to climb up to FL360 and start up all the instruments")
c_meteor = c_climb.course(90, 7e3).assign(label="c_meteor", fl=410)
wp_meteor = LatLon(bco.lat, c_meteor.lon - 0.665)
lon_min, lon_max, lat_min, lat_max = -60, -57.5, 12.4, 14
plt.figure(figsize = (14, 8))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([lon_min, lon_max, lat_min, lat_max], crs=ccrs.PlateCarree())
ax.coastlines(alpha=1.0)
ax.gridlines(draw_labels=True, dms=True, x_inline=False, y_inline=False, alpha = 0.25)
plan = FlightPlan([
tbpb.assign(time="2024-09-23T11:04:16Z"),
wp_meteor.assign(fl=150, label="wp_meteor"),
IntoCircle(c_climb, radius=65e3, angle=350),
IntoCircle(c_meteor, radius=radius_meteor, angle=360),
], aircraft="HALO")
plot_path(plan, ax, color="C1")
plt.scatter(wp_meteor.lon, wp_meteor.lat, color="C2", marker="o")
plan.show_details()
Detailed Overview:
TBPB N13 04.48, W059 29.55, FL000, 11:04:16 UTC,
to wp_meteor N13 09.76, W059 25.15, FL150, 11:05:25 UTC,
circle around c_climb N13 09.76, W058 49.12, FL150, 11:05:26 UTC - 11:42:02 UTC, radius: 35 nm, 350° CW, enter from west, circle to climb up to FL360 and start up all the instruments
circle around c_meteor N13 09.76, W058 45.25, FL410, 11:42:03 UTC - 12:14:12 UTC, radius: 39 nm, 360° CW, enter from west
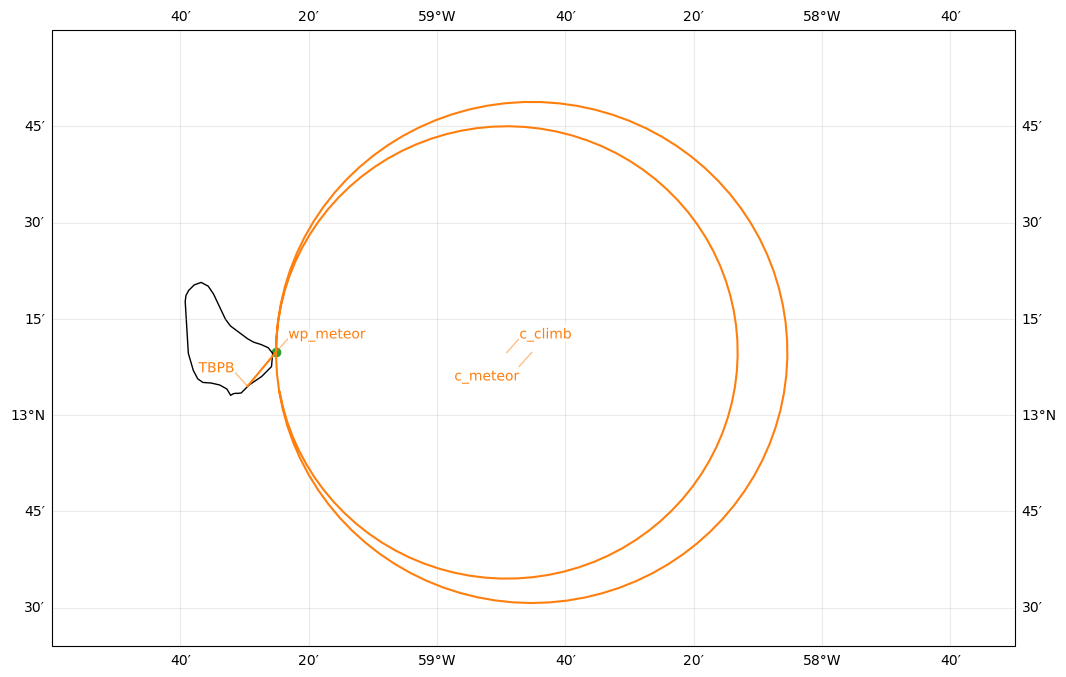
Flight path#
Show code cell source
c_climb = bco.assign(lon=bco.lon+0.61, label="c_climb", fl=150)
wp_meteor = LatLon(bco.lat, c_meteor.lon - 0.665)
c_meteor = c_climb.course(90, 7e3).assign(label="c_meteor", fl=410)
c_south = LatLon(lat=10.5, lon=-46).assign(label = "c_south", fl=450)
ec_north = point_on_track(ec_track, lat=12.9).assign(label="ec_north")
ec_under = point_on_track(ec_track, lat=11.025, with_time=True).assign(label="ec_under", note="meet EarthCARE")
ec_south = point_on_track(ec_track, lat=9.15).assign(label="ec_south")
waypoints = [
tbpb,
wp_meteor.assign(fl=150, label="wp_meteor"),
IntoCircle(c_climb, radius=65e3, angle=360),
IntoCircle(c_meteor, radius=radius_meteor, angle=360),
IntoCircle(tbpb.towards(c_south, fraction=.5).assign(fl=430, label="c_mid"), radius, -360),
IntoCircle(tbpb.towards(c_south, fraction=.75).assign(fl=450, label="c_ec"), radius, -360),
IntoCircle(c_south.assign(fl=450), radius, -360),
ec_south.assign(fl=450),
ec_under.assign(fl=450),
ec_north.assign(fl=450),
point_on_track(ec_track, lat=11.1).assign(fl=450, label="wp_home"),
IntoCircle(tbpb.towards(c_south, fraction=.25).assign(fl=450, label="c_west"), radius, -360),
wp_meteor.assign(fl=150, label="wp_meteor"),
tbpb,
]
plan = FlightPlan(waypoints, flight_index, crew=crew, aircraft="HALO")
print(f"Take-off: {plan.takeoff_time:%H:%M %Z}\nLanding: {plan.landing_time:%H:%M %Z}\nDuration: {plan.duration}\n")
Take-off: 11:04 UTC
Landing: 20:16 UTC
Duration: 9:12:28.486656
Plotting flight path on CWV#
Show code cell source
lon_min, lon_max, lat_min, lat_max = -65, -40, 5, 17 #-65, -20, 0, 20
plt.figure(figsize = (14, 8))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.set_extent([lon_min, lon_max, lat_min, lat_max], crs=ccrs.PlateCarree())
ax.coastlines(alpha=1.0)
ax.gridlines(draw_labels=True, dms=True, x_inline=False, y_inline=False, alpha = 0.25)
plt.title(f"{flight_time}\n(CWV forecast issued on {ifs_fcst_time})")
plot_fir(ax=ax)
plot_cwv(cwv_flight_time, levels = [48.0, 50.0, 52.0, 54.0, 56.0, 58.0, 60.0], ax=ax)
plt.plot(ec_track.lon, ec_track.lat, c='k', ls='dotted')
plot_path(plan, ax, color="C1")
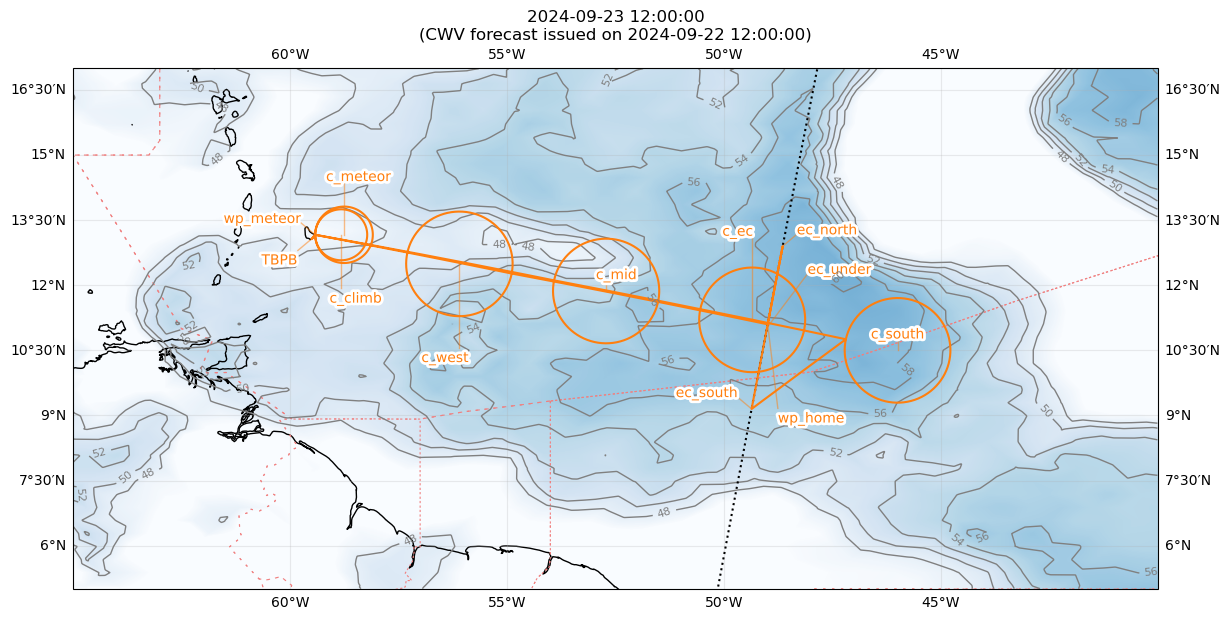
Description#
we start our flight with a small circle to climb up to FL410 (c_climb)and ramp up all instruments east of Barbados.
we continue with a circle of 72km radius c_meteor in coordination with Meteor measurements (within the SEA-POL scan range) and with dropping 12 sondes. Meteor plans to sit at the western most point of the circle and we might overpass it twice.
we continue circling our way east c_mid, c_ec, c_south and with 12 sondes per circle.
we will next go on the EarthCARE track entering it at ec_south, meet EC at 17:22:37 UTC at ec_under, and continue to ec_north. A procedure turn will bring us back to on the EC track to the waypoint wp_home
we head home and do one last circle c_west on the way, again with 12 sondes.
In total we plan to throw 5*12=60 sondes.
Waypoint coordinates and times#
Show code cell source
plan.show_details()
plan.export()
Detailed Overview:
TBPB N13 04.48, W059 29.55, FL000, 11:04:16 UTC,
to wp_meteor N13 09.76, W059 25.15, FL150, 11:05:25 UTC,
circle around c_climb N13 09.76, W058 49.12, FL150, 11:05:26 UTC - 11:43:05 UTC, radius: 35 nm, 360° CW, enter from west
circle around c_meteor N13 09.76, W058 45.25, FL410, 11:43:05 UTC - 12:15:15 UTC, radius: 39 nm, 360° CW, enter from west
circle around c_mid N11 52.07, W052 42.88, FL430, 12:58:22 UTC - 13:57:23 UTC, radius: 72 nm, 360° CCW, enter from west
circle around c_ec N11 12.17, W049 20.97, FL450, 14:23:40 UTC - 15:22:12 UTC, radius: 72 nm, 360° CCW, enter from west
circle around c_south N10 30.00, W046 00.00, FL450, 15:48:22 UTC - 16:46:55 UTC, radius: 72 nm, 360° CCW, enter from west
to ec_south N09 09.00, W049 21.77, FL450, 17:07:41 UTC,
to ec_under N11 01.50, W049 00.37, FL450, 17:22:26 UTC, meet EarthCARE
to ec_north N12 54.00, W048 38.80, FL450, 17:37:11 UTC,
to wp_home N11 06.00, W048 59.52, FL450, 17:51:21 UTC,
circle around c_west N12 29.55, W056 05.74, FL450, 18:37:12 UTC - 19:35:45 UTC, radius: 72 nm, 360° CCW, enter from east
to wp_meteor N13 09.76, W059 25.15, FL150, 20:15:35 UTC,
to TBPB N13 04.48, W059 29.55, FL000, 20:16:44 UTC,