Flight plan - HALO-20240916a#
ec_under ec_track c_north c_mid c_south pace_under curtainCrew#
Job |
Name |
---|---|
PI |
Konstantin Krüger |
WALES |
Dimitra Kouklaki |
HAMP |
Georgios Dekoutsidis |
Dropsondes |
Nina Robbins |
Smart/VELOX |
Michael Schäfer |
SpecMACS |
Anja Stallmach |
Flight Documentation |
Manfred Wendisch |
Ground contact |
Raphaela Vogel |
Flight plan#
/home/runner/miniconda3/envs/orcestra_book/lib/python3.12/site-packages/orcestra/sat.py:195: UserWarning: You are using an old forecast (issued on 2024-09-15) for EARTHCARE on 2024-09-16! The newest forecast issued so far was issued on 2024-09-16. It's a PRE forecast.
warnings.warn(
Flight index: HALO-20240916a
Take-off: 11:54 UTC
Landing: 20:48 UTC
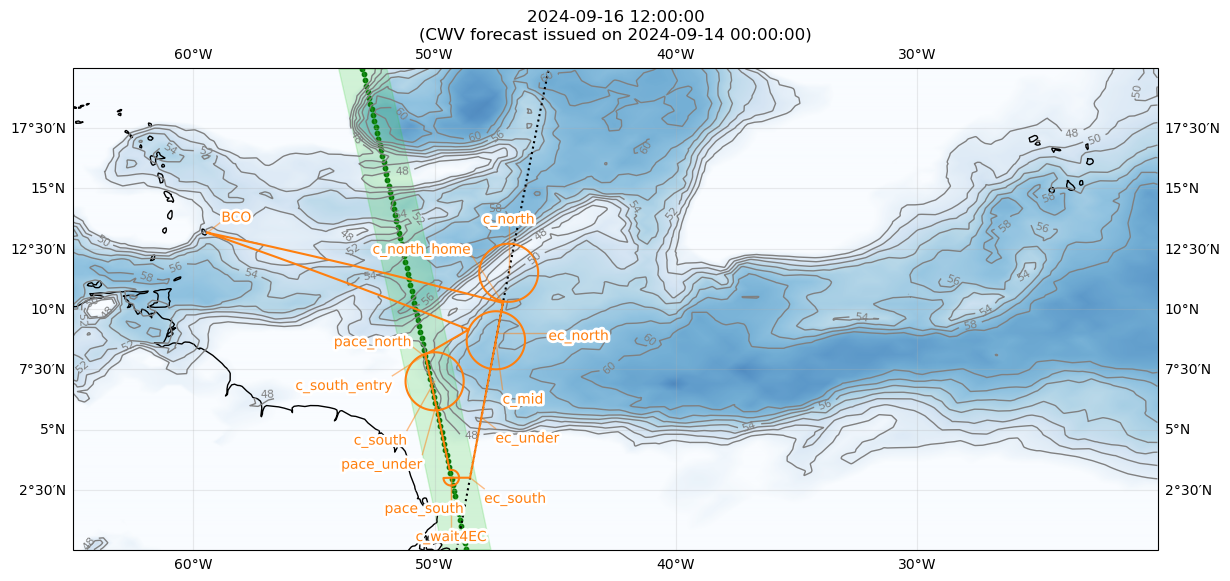
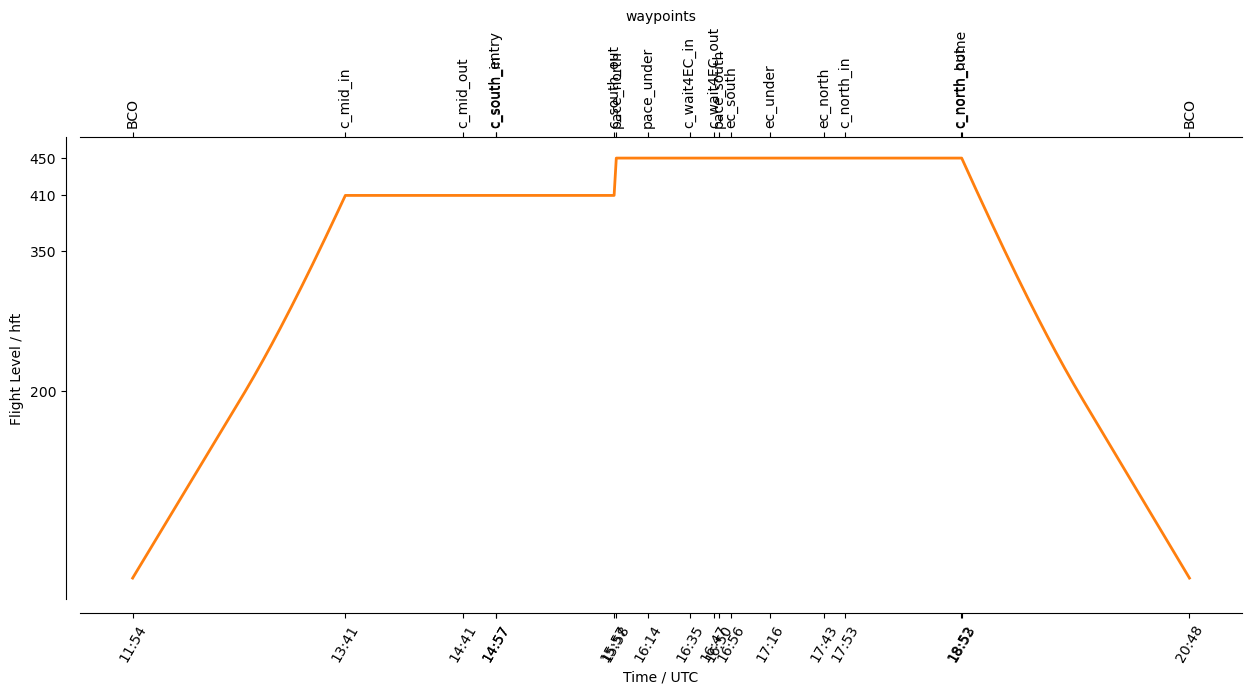
Detailed Overview:
BCO N13 09.76, W059 25.72, FL000, 11:54:14 UTC,
circle around c_mid N08 42.00, W047 27.59, FL410, 13:41:41 UTC - 14:41:15 UTC, radius: 72 nm, 360° CCW, enter from west
to c_south_entry N08 06.00, W050 23.26, FL410, 14:57:34 UTC,
circle around c_south N07 00.00, W050 00.00, FL410, 14:57:52 UTC - 15:57:26 UTC, radius: 72 nm, 360° CCW, enter from north
to pace_north N08 00.00, W050 21.99, FL450, 15:58:32 UTC,
to pace_under N06 00.00, W049 56.50, FL450, 16:14:20 UTC,
circle around c_wait4EC N03 00.00, W049 18.56, FL450, 16:35:31 UTC - 16:47:49 UTC, radius: 19 nm, 280° CW, enter from north
to pace_south N03 00.00, W049 18.56, FL450, 16:50:20 UTC,
to ec_south N03 00.00, W048 31.75, FL450, 16:56:24 UTC,
to ec_under N05 30.00, W048 03.72, FL450, 17:16:03 UTC, meet EarthCARE
to ec_north N09 00.00, W047 24.18, FL450, 17:43:35 UTC,
circle around c_north N11 30.00, W046 55.64, FL450, 17:53:56 UTC - 18:52:29 UTC, radius: 72 nm, 360° CW, enter from south
to c_north_home N10 15.00, W047 09.95, FL450, 18:53:00 UTC,
to BCO N13 09.76, W059 25.72, FL000, 20:48:03 UTC,